Welcome in third part of my tutorial!
Today we are going to learn common thing while code refactor – class movement.
What I always did was to manually move the file in the Finder and then changed it’s namespace in the IDE. Finally I had to search whole project for class name etc. But PhpStorm can do all of that with one simple action.
I’m going to move the class \app\models\User to \common\models\User (Yii2 project).
Let me show you the beginning of my class:
<?php
namespace app\models;
use yii\base\Exception;
use yii\base\Model;
use yii\base\NotSupportedException;
use yii\web\IdentityInterface;
class User extends Model implements IdentityInterface
{
First thing what you need to do is to move the cursor into class name and open refactor menu (right click -> refactor, or press ctrl + t) and choose Move.
Fill the target namespace. I always check two checkboxes, Search in comments and string and Search for text occurrences. This will allows us to refactor the PHPDoc, comments and other places where the class name exists.
Before pressing the Refactor button, I recommend to Preview the changes. All found places you will see on the list like this:
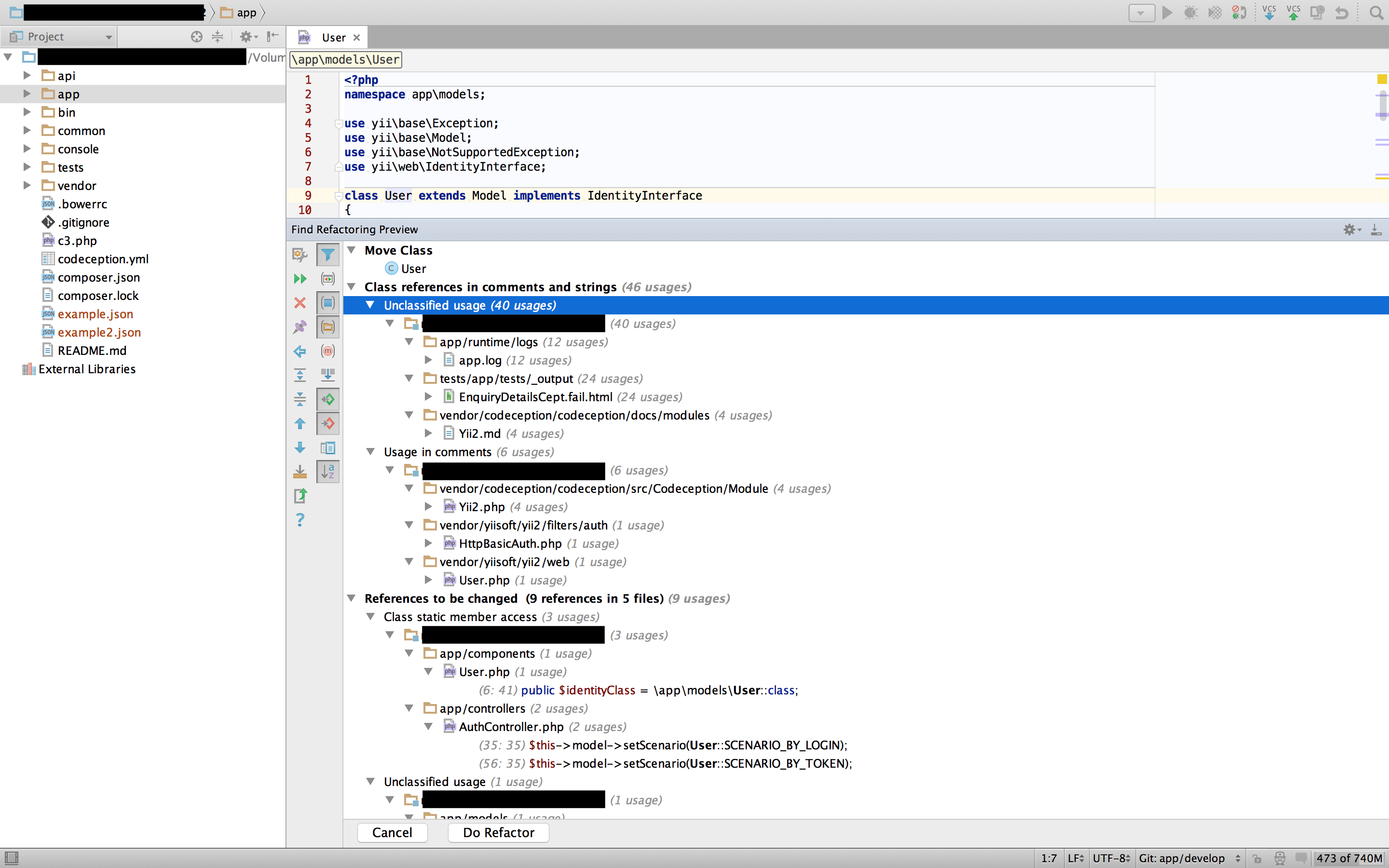
With second mouse button you can exclude results from refactoring. When you’re done, press Do Refactor. Now run your tests. Everything should work like as a charm!
Thank you for reading. Have a good day!